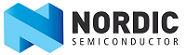 |
Developing with ZBOSS SDK for Zigbee
|
|
#define | ZB_ZCL_READ_REP_CFG_REQ_SIZE sizeof(zb_zcl_read_reporting_cfg_req_t) |
| Minimal size of Write attribute request, it will be more if attr_value size is more than 1 byte.
|
|
#define | ZB_ZCL_GENERAL_GET_NEXT_READ_REP_CFG_REQ(data_buf, rep_cfg_req, rslt) |
| Parses read reporting configuration request and returns next Read reporting configuration attribute record or NULL if there is no more data. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_READ_REPORTING_CONFIGURATION_SRV_REQ(buffer, ptr, def_resp) |
| Initialize Read reporting configuration command (report send case) More...
|
|
#define | ZB_ZCL_GENERAL_INIT_READ_REPORTING_CONFIGURATION_CLI_REQ(buffer, ptr, def_resp) |
| Initialize Read reporting configuration command (report receive case) More...
|
|
#define | ZB_ZCL_GENERAL_ADD_SEND_READ_REPORTING_CONFIGURATION_REQ(ptr, attr_id) |
| Add Read reporting configuration record to command payload (report send case) More...
|
|
#define | ZB_ZCL_GENERAL_ADD_RECV_READ_REPORTING_CONFIGURATION_REQ(ptr, attr_id) |
| Add Read reporting configuration record to command payload (report receive case) More...
|
|
#define | ZB_ZCL_GENERAL_SEND_READ_REPORTING_CONFIGURATION_REQ( buffer, ptr, addr, dst_addr_mode, dst_ep, ep, prfl_id, cluster_id, cb) |
| Sends Read reporting configuration command. More...
|
|
#define | ZB_ZCL_GENERAL_GET_READ_REPORTING_CONFIGURATION_RES(data_buf, read_rep_conf_res) |
| Parses Read reporting configuration response and returns next read reporting configuration record or NULL if there is no more data. More...
|
|
Most of actions related to the read attribute reporting configuration are implemented in ZCL internals. Read reporting configuration command is described in ZCL spec, subclause 2.4.9.
◆ ZB_ZCL_GENERAL_ADD_RECV_READ_REPORTING_CONFIGURATION_REQ
#define ZB_ZCL_GENERAL_ADD_RECV_READ_REPORTING_CONFIGURATION_REQ |
( |
|
ptr, |
|
|
|
attr_id |
|
) |
| |
Value:{ \
ZB_ZCL_PACKET_PUT_DATA16_VAL(ptr, (attr_id)); \
}
Add Read reporting configuration record to command payload (report receive case)
- Parameters
-
ptr | - command buffer pointer |
attr_id | - attribute identifier |
◆ ZB_ZCL_GENERAL_ADD_SEND_READ_REPORTING_CONFIGURATION_REQ
#define ZB_ZCL_GENERAL_ADD_SEND_READ_REPORTING_CONFIGURATION_REQ |
( |
|
ptr, |
|
|
|
attr_id |
|
) |
| |
Value:{ \
ZB_ZCL_PACKET_PUT_DATA16_VAL(ptr, (attr_id)); \
}
Add Read reporting configuration record to command payload (report send case)
- Parameters
-
ptr | - command buffer pointer |
attr_id | - attribute identifier |
◆ ZB_ZCL_GENERAL_GET_NEXT_READ_REP_CFG_REQ
#define ZB_ZCL_GENERAL_GET_NEXT_READ_REP_CFG_REQ |
( |
|
data_buf, |
|
|
|
rep_cfg_req, |
|
|
|
rslt |
|
) |
| |
Value: { \
zb_zcl_read_reporting_cfg_req_t *cfg_req; \
if (cfg_req) \
{ \
rep_cfg_req.direction = cfg_req -> direction; \
rep_cfg_req.attr_id = cfg_req -> attr_id; \
ZB_ZCL_HTOLE16_INPLACE(&(read_rep_cfg_req).attr_id); \
} \
else \
{ \
} \
}
Parses read reporting configuration request and returns next Read reporting configuration attribute record or NULL if there is no more data.
If request contains invalid data, NULL is returned.
- Parameters
-
data_buf | - ID zb_bufid_t of a buffer containing read reporting configuration request data |
rep_cfg_req | - out pointer to zb_zcl_read_attr_req_t, containing read reporting configuration request |
rslt | - returns TRUE if record exist and FALSE if not |
- Note
- data_buf buffer should contain read reporting configuration request payload, without ZCL header. Each parsed read reporting configuration request is extracted from initial data_buf buffer
◆ ZB_ZCL_GENERAL_GET_READ_REPORTING_CONFIGURATION_RES
#define ZB_ZCL_GENERAL_GET_READ_REPORTING_CONFIGURATION_RES |
( |
|
data_buf, |
|
|
|
read_rep_conf_res |
|
) |
| |
Parses Read reporting configuration response and returns next read reporting configuration record or NULL if there is no more data.
If response contains invalid data, NULL is returned.
- Parameters
-
data_buf | - ID zb_bufid_t of a buffer containing Configure reporting response data |
read_rep_conf_res | - out pointer to zb_zcl_read_reporting_cfg_rsp_t, containing read reporting configuration record |
- Note
- data_buf buffer should contain Read reporting configuration, without ZCL header. Each parsed Read reporting configuration record is extracted from initial data_buf buffer
◆ ZB_ZCL_GENERAL_INIT_READ_REPORTING_CONFIGURATION_CLI_REQ
#define ZB_ZCL_GENERAL_INIT_READ_REPORTING_CONFIGURATION_CLI_REQ |
( |
|
buffer, |
|
|
|
ptr, |
|
|
|
def_resp |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_REQ_FRAME_CONTROL_A( \
}
Initialize Read reporting configuration command (report receive case)
- Parameters
-
buffer | to put packet to |
ptr | - command buffer pointer |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_INIT_READ_REPORTING_CONFIGURATION_SRV_REQ
#define ZB_ZCL_GENERAL_INIT_READ_REPORTING_CONFIGURATION_SRV_REQ |
( |
|
buffer, |
|
|
|
ptr, |
|
|
|
def_resp |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_REQ_FRAME_CONTROL_A( \
}
Initialize Read reporting configuration command (report send case)
- Parameters
-
buffer | to put packet to |
ptr | - command buffer pointer |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_SEND_READ_REPORTING_CONFIGURATION_REQ
#define ZB_ZCL_GENERAL_SEND_READ_REPORTING_CONFIGURATION_REQ |
( |
|
buffer, |
|
|
|
ptr, |
|
|
|
addr, |
|
|
|
dst_addr_mode, |
|
|
|
dst_ep, |
|
|
|
ep, |
|
|
|
prfl_id, |
|
|
|
cluster_id, |
|
|
|
cb |
|
) |
| |
Value:{ \
ZB_ZCL_FINISH_PACKET(buffer, ptr) \
ZB_ZCL_SEND_COMMAND_SHORT(buffer, addr, dst_addr_mode, dst_ep, ep, prfl_id, cluster_id, cb); \
}
Sends Read reporting configuration command.
- Parameters
-
buffer | to put data to |
ptr | - pointer to the memory area to put data to |
addr | - address to send packet to |
dst_addr_mode | - addressing mode |
dst_ep | - destination endpoint |
ep | - sending endpoint |
prfl_id | - profile identifier |
cluster_id | - cluster identifier |
cb | - callback for getting command send status |
◆ zb_zcl_read_reporting_cfg_req_t
Format of the Attribute Status Record Field Figure 2.20 in ZCL spec. NOTE: it can be various number of attribute status record fields in Read reporting configuration request
◆ zb_zcl_read_reporting_cfg_rsp_t
Format of the Attribute Reporting Configuration Record Field Figure 2.22 in ZCL spec. NOTE: it can be various number of attribute recording configuration record fields in Read reporting configuration response
#define ZB_ZCL_START_PACKET(zbbuf)
Initializes zb_buf_t buffer and returns pointer to the beginning of array.
Definition: zb_zcl_common.h:1354
#define ZB_ZCL_READ_REP_CFG_REQ_SIZE
Minimal size of Write attribute request, it will be more if attr_value size is more than 1 byte.
Definition: zb_zcl_commands.h:1754
@ ZB_TRUE
Definition: zb_types.h:130
#define ZB_ZCL_GET_SEQ_NUM()
Return next sequence number for ZCL frame.
Definition: zb_zcl_common.h:1272
@ ZB_ZCL_FRAME_DIRECTION_TO_SRV
Definition: zb_zcl_common.h:895
#define zb_buf_cut_left(buf, size)
Definition: zboss_api_buf.h:485
@ ZB_ZCL_CONFIGURE_REPORTING_RECV_REPORT
Definition: zb_zcl_commands.h:1429
@ ZB_FALSE
Definition: zb_types.h:129
Definition: zb_zcl_commands.h:1738
@ ZB_ZCL_CONFIGURE_REPORTING_SEND_REPORT
Definition: zb_zcl_commands.h:1428
#define zb_buf_begin(buf)
Definition: zboss_api_buf.h:331
@ ZB_ZCL_FRAME_DIRECTION_TO_CLI
Definition: zb_zcl_common.h:897
@ ZB_ZCL_NOT_MANUFACTURER_SPECIFIC
Standard profile command.
Definition: zb_zcl_common.h:867
@ ZB_ZCL_CMD_READ_REPORT_CFG
Definition: zb_zcl_commands.h:69
#define zb_buf_len(buf)
Definition: zboss_api_buf.h:378