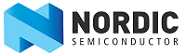 |
Developing with ZBOSS for Zigbee
|
|
#define | ZB_ZCL_GENERAL_GET_NEXT_READ_ATTR_RES(data_buf, read_attr_resp) |
| Parses Read attribute response and returns next Read attribute status record or NULL if there is no more data. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ(buffer, cmd_ptr, def_resp) |
| Initialize Read attribute command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ_A(buffer, cmd_ptr, direction, def_resp) |
| Initialize Read Attribute Request command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ_MANUF(buffer, cmd_ptr, direction, def_resp, manuf_code) |
| Initialize Read Attribute Request command with manufacturer code. More...
|
|
#define | ZB_ZCL_GENERAL_ADD_ID_READ_ATTR_REQ(cmd_ptr, attr_id) |
| Add attribute id to command payload. More...
|
|
#define | ZB_ZCL_GENERAL_SEND_READ_ATTR_REQ(buffer, cmd_ptr, addr, dst_addr_mode, dst_ep, ep, profile_id, cluster_id, cb) |
| Sends Read attribute command. More...
|
|
#define | ZB_ZCL_GENERAL_GET_READ_ATTR_REQ(_data_buf, _read_attr_req) |
| Parses Read attribute request and returns next Read attribute record or NULL if there is no more data. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_READ_ATTR_RESP(_buffer, _cmd_ptr, _seq) |
| Initialize Read Attribute Response command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_READ_ATTR_RESP_EXT(_buffer, _cmd_ptr, _direction, _seq, _is_manuf, _manuf_id) |
| Initialize Read Attribute Response command. More...
|
|
#define | ZB_ZCL_GENERAL_SEND_READ_ATTR_RESP( _buffer, _cmd_ptr, _addr, _dst_addr_mode, _dst_ep, _ep, _profile_id, _cluster_id, _cb) |
| Send Read attribute response command. More...
|
|
Read attributes command described in ZCL spec, subclauses 2.4.1 and 2.4.2.
Read attributes request command can be formed and sent as in following snippet:
Read attributes response can be parsed as:
until allocated buffer space exceeds.
Read attributes request parsing and response filling and sending is implemented in ZCL library internal functions.
◆ ZB_ZCL_GENERAL_ADD_ID_READ_ATTR_REQ
#define ZB_ZCL_GENERAL_ADD_ID_READ_ATTR_REQ |
( |
|
cmd_ptr, |
|
|
|
attr_id |
|
) |
| |
Value:{ \
ZB_ZCL_PACKET_PUT_DATA16_VAL(cmd_ptr, (attr_id)); \
}
Add attribute id to command payload.
- Parameters
-
cmd_ptr | - command buffer pointer |
attr_id | - attribute ID |
◆ ZB_ZCL_GENERAL_GET_NEXT_READ_ATTR_RES
#define ZB_ZCL_GENERAL_GET_NEXT_READ_ATTR_RES |
( |
|
data_buf, |
|
|
|
read_attr_resp |
|
) |
| |
Parses Read attribute response and returns next Read attribute status record or NULL if there is no more data.
If response contains invalid data, NULL is returned.
- Parameters
-
data_buf | - ID zb_bufid_t of a buffer containing read attribute response data |
read_attr_resp | - out pointer to zb_zcl_read_attr_res_t, containing Read attribute status record |
- Note
- data_buf buffer should contain Read attribute response payload, without ZCL header. Each parsed Read attribute status record is extracted from initial data_buf buffer
◆ ZB_ZCL_GENERAL_GET_READ_ATTR_REQ
#define ZB_ZCL_GENERAL_GET_READ_ATTR_REQ |
( |
|
_data_buf, |
|
|
|
_read_attr_req |
|
) |
| |
Value:{ \
\
if ((_read_attr_req)) \
{ \
ZB_ZCL_HTOLE16_INPLACE(&(_read_attr_req)->attr_id); \
} \
}
Parses Read attribute request and returns next Read attribute record or NULL if there is no more data.
If request contains invalid data, NULL is returned.
- Parameters
-
_data_buf | - ID zb_bufid_t of a buffer containing write attribute request data |
_read_attr_req | - out pointer to zb_zcl_read_attr_req_t, containing Read attribute record out value direct into data_buf. Do not change data_buf before finish work with read_attr_req |
- Note
- data_buf buffer should contain Read attribute request payload, without ZCL header. Each parsed Read attribute record is extracted from initial data_buf buffer
◆ ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ
#define ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
def_resp |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_REQ_FRAME_CONTROL(cmd_ptr, def_resp); \
}
Initialize Read attribute command.
- Parameters
-
buffer | to put packet to |
cmd_ptr | - command buffer pointer |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ_A
#define ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ_A |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
direction, |
|
|
|
def_resp |
|
) |
| |
Value:
Initialize Read Attribute Request command.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
direction | - direction of command (see zcl_frame_direction) |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ_MANUF
#define ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ_MANUF |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
direction, |
|
|
|
def_resp, |
|
|
|
manuf_code |
|
) |
| |
Value:
Initialize Read Attribute Request command with manufacturer code.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
direction | - direction of command (see zcl_frame_direction) |
def_resp | - enable/disable default response |
manuf_code | - manufacturer specific code |
◆ ZB_ZCL_GENERAL_INIT_READ_ATTR_RESP
#define ZB_ZCL_GENERAL_INIT_READ_ATTR_RESP |
( |
|
_buffer, |
|
|
|
_cmd_ptr, |
|
|
|
_seq |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_RESP_FRAME_CONTROL((_cmd_ptr)); \
}
Initialize Read Attribute Response command.
- Parameters
-
_buffer | - buffer to store command data |
_cmd_ptr | - pointer to a command data memory |
_seq | - command sequence |
◆ ZB_ZCL_GENERAL_INIT_READ_ATTR_RESP_EXT
#define ZB_ZCL_GENERAL_INIT_READ_ATTR_RESP_EXT |
( |
|
_buffer, |
|
|
|
_cmd_ptr, |
|
|
|
_direction, |
|
|
|
_seq, |
|
|
|
_is_manuf, |
|
|
|
_manuf_id |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_RESP_FRAME_CONTROL_A((_cmd_ptr), (_direction), (_is_manuf)); \
}
Initialize Read Attribute Response command.
- Parameters
-
_buffer | - buffer to store command data |
_cmd_ptr | - pointer to a command data memory |
_direction | - direction of command (see zcl_frame_direction) |
_seq | - command sequence |
_is_manuf | - whether command is manufacturer specific |
_manuf_id | - manufacturer ID (needed if _is_manuf is set) |
◆ ZB_ZCL_GENERAL_SEND_READ_ATTR_REQ
#define ZB_ZCL_GENERAL_SEND_READ_ATTR_REQ |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
addr, |
|
|
|
dst_addr_mode, |
|
|
|
dst_ep, |
|
|
|
ep, |
|
|
|
profile_id, |
|
|
|
cluster_id, |
|
|
|
cb |
|
) |
| |
Value:
ZB_ZCL_SEND_COMMAND_SHORT(buffer, addr, dst_addr_mode, dst_ep, ep, profile_id, cluster_id, cb);
Sends Read attribute command.
- Parameters
-
buffer | to place data to |
cmd_ptr | - pointer to the memory area after the command data end |
addr | - address to send packet to |
dst_addr_mode | - addressing mode |
dst_ep | - destination endpoint |
ep | - sending endpoint |
profile_id | - profile identifier |
cluster_id | - cluster identifier |
cb | - callback for getting command send status |
◆ ZB_ZCL_GENERAL_SEND_READ_ATTR_RESP
#define ZB_ZCL_GENERAL_SEND_READ_ATTR_RESP |
( |
|
_buffer, |
|
|
|
_cmd_ptr, |
|
|
|
_addr, |
|
|
|
_dst_addr_mode, |
|
|
|
_dst_ep, |
|
|
|
_ep, |
|
|
|
_profile_id, |
|
|
|
_cluster_id, |
|
|
|
_cb |
|
) |
| |
Value:{ \
ZB_ZCL_FINISH_PACKET((_buffer), (_cmd_ptr)) \
ZB_ZCL_SEND_COMMAND_SHORT((_buffer), (_addr), (_dst_addr_mode), (_dst_ep), (_ep), \
(_profile_id), (_cluster_id), (_cb)); \
}
Send Read attribute response command.
- Parameters
-
_buffer | - buffer to store command data |
_cmd_ptr | - pointer to a command data memory |
_addr | - address to send packet to |
_dst_addr_mode | - addressing mode |
_dst_ep | - destination endpoint |
_ep | - sending endpoint |
_profile_id | - profile identifier |
_cluster_id | - cluster identifier |
_cb | - callback for getting command send status |
◆ zb_zcl_read_attr_req_t
ZCL Read Attribute Command frame.
- See also
- ZCL spec, zb_zcl_read_attr 2.4.1 Read Attributes Command
- Note
- Command frame contains variable number of parameters
◆ zb_zcl_read_attr_res_t
ZCL Read Attribute Response Command frame.
- See also
- ZCL spec, zb_zcl_read_attr 2.4.2 Read Attributes Response Command
- Note
- Command frame contains variable number of parameters. Also, based on status value attr_type and attr_value maybe absent.
#define ZB_AF_HA_PROFILE_ID
Definition: zboss_api_af.h:287
#define ZB_ZCL_GENERAL_SEND_READ_ATTR_REQ(buffer, cmd_ptr, addr, dst_addr_mode, dst_ep, ep, profile_id, cluster_id, cb)
Sends Read attribute command.
Definition: zb_zcl_commands.h:715
#define ZB_ZCL_START_PACKET(zbbuf)
Initializes zb_buf_t buffer and returns pointer to the beginning of array.
Definition: zb_zcl_common.h:1530
#define ZB_ZCL_ENABLE_DEFAULT_RESPONSE
Definition: zb_zcl_common.h:1026
#define ZB_ZCL_CMD_READ_ATTRIB
Definition: zb_zcl_commands.h:66
#define ZB_ZCL_CMD_READ_ATTRIB_RESP
Definition: zb_zcl_commands.h:67
#define ZB_ZCL_GET_SEQ_NUM()
Return next sequence number for ZCL frame.
Definition: zb_zcl_common.h:1450
#define ZB_ZCL_NOT_MANUFACTURER_SPECIFIC
Definition: zb_zcl_common.h:1000
#define zb_buf_cut_left(buf, size)
Definition: zboss_api_buf.h:464
#define ZB_TRUE
Definition: zb_types.h:375
#define ZB_ZCL_CLUSTER_ID_BINARY_INPUT
Definition: zb_zcl_common.h:221
#define ZB_ZCL_GENERAL_GET_NEXT_READ_ATTR_RES(data_buf, read_attr_resp)
Parses Read attribute response and returns next Read attribute status record or NULL if there is no m...
Definition: zb_zcl_commands.h:619
#define zb_buf_begin(buf)
Definition: zboss_api_buf.h:343
#define ZB_ZCL_MANUFACTURER_SPECIFIC
Definition: zb_zcl_common.h:1003
#define ZB_ZCL_GENERAL_ADD_ID_READ_ATTR_REQ(cmd_ptr, attr_id)
Add attribute id to command payload.
Definition: zb_zcl_commands.h:699
ZCL Read Attribute Command frame.
Definition: zb_zcl_commands.h:583
@ ZB_ZCL_ATTR_BINARY_INPUT_PRESENT_VALUE_ID
PresentValue attribute.
Definition: zb_zcl_binary_input.h:72
#define zb_buf_len(buf)
Definition: zboss_api_buf.h:361
#define ZB_ZCL_GENERAL_INIT_READ_ATTR_REQ(buffer, cmd_ptr, def_resp)
Initialize Read attribute command.
Definition: zb_zcl_commands.h:657
struct zb_zcl_read_attr_req_s zb_zcl_read_attr_req_t
ZCL Read Attribute Command frame.
#define ZB_ZCL_FINISH_PACKET(zbbuf, ptr)
Definition: zb_zcl_common.h:1830