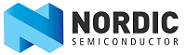 |
Developing with ZBOSS for Zigbee
|
|
#define | ZB_ZCL_GENERAL_GET_NEXT_WRITE_ATTR_REQ(data_ptr, data_len, write_attr_req) |
| Parses Write attribute request and returns next Write attribute record or NULL if there is no more data. More...
|
|
#define | ZB_ZCL_GET_NEXT_WRITE_ATTR_RES(data_buf, write_attr_res) |
| Parses Write attribute response and returns next Write attribute status or NULL if there is no more data. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_BY_TYPE(buffer, cmd_ptr, def_resp, write_attr_type) |
| Initialize Write attribute command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ(buffer, cmd_ptr, def_resp) |
| Initialize Write attribute command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_NO_RESP(buffer, cmd_ptr, def_resp) |
| Initialize Write Attribute No Response command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_UNDIV(buffer, cmd_ptr, def_resp) |
| Initialize Write Attribute Undivided command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_A(buffer, cmd_ptr, direction, def_resp) |
| Initialize Write attribute command. More...
|
|
#define | ZB_ZCL_GENERAL_ADD_VALUE_WRITE_ATTR_REQ(cmd_ptr, attr_id, attr_type, attr_val) |
| Add attribute value to command payload. More...
|
|
#define | ZB_ZCL_GENERAL_SEND_WRITE_ATTR_REQ( buffer, cmd_ptr, addr, dst_addr_mode, dst_ep, ep, profile_id, cluster_id, cb) |
| Send Write attribute command. More...
|
|
#define | ZB_ZCL_GENERAL_SEND_WRITE_ATTRS_REQ( buffer, addr, addr_mode, dst_ep, ep, prof_id, cluster_id) |
| Send "write attributes" request. deprecate. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_WRITE_ATTR_RESP(_buffer, _cmd_ptr, _seq) |
| Initialize Write attribute response command. More...
|
|
#define | ZB_ZCL_GENERAL_INIT_WRITE_ATTR_RESP_EXT(_buffer, _cmd_ptr, _direction, _seq, _is_manuf, _manuf_id) |
| Initialize Write attribute response command. More...
|
|
#define | ZB_ZCL_GENERAL_SUCCESS_WRITE_ATTR_RESP(_cmd_ptr) |
| Add Success status value to Write attribute response command payload. More...
|
|
#define | ZB_ZCL_GENERAL_SEND_WRITE_ATTR_RESP( _buffer, _cmd_ptr, _addr, _dst_addr_mode, _dst_ep, _ep, _profile_id, _cluster_id, _cb) |
| Send Write attribute response command. More...
|
|
Both write attributes request and response commands have variable-length payload.
Write attributes request can be filled as following:
data_buf,
ptr,
dst_addr,
dst_ep,
src_ep,
NULL);
On the server side, this packet could be parsed in the following manner:
...
do
{
if (write_attr_req)
{
process write attribute request record
}
}
while(write_attr_req);
Response sending and parsing could be done in the same manner.
◆ ZB_ZCL_GENERAL_ADD_VALUE_WRITE_ATTR_REQ
#define ZB_ZCL_GENERAL_ADD_VALUE_WRITE_ATTR_REQ |
( |
|
cmd_ptr, |
|
|
|
attr_id, |
|
|
|
attr_type, |
|
|
|
attr_val |
|
) |
| |
Value:{ \
ZB_ZCL_PACKET_PUT_DATA16_VAL(cmd_ptr, (attr_id)); \
ZB_ZCL_PACKET_PUT_DATA8(cmd_ptr, (attr_type)); \
}
Add attribute value to command payload.
- Parameters
-
cmd_ptr | - pointer to a command data memory |
attr_id | - attribute identifier |
attr_type | - attribute type |
attr_val | - pointer to attribute data value |
◆ ZB_ZCL_GENERAL_GET_NEXT_WRITE_ATTR_REQ
#define ZB_ZCL_GENERAL_GET_NEXT_WRITE_ATTR_REQ |
( |
|
data_ptr, |
|
|
|
data_len, |
|
|
|
write_attr_req |
|
) |
| |
Parses Write attribute request and returns next Write attribute record or NULL if there is no more data.
If request contains invalid data, NULL is returned.
- Parameters
-
data_ptr | - pointer to the data of a zb_bufid_t buffer containing write attribute request data |
data_len | - variable containing length of a zb_bufid_t buffer |
write_attr_req | - out pointer to zb_zcl_write_attr_req_t, containing Write attribute record |
- Note
- buffer data by data_ptr should contain Write attribute request payload, without ZCL header.
◆ ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
def_resp |
|
) |
| |
Value:
Initialize Write attribute command.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_A
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_A |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
direction, |
|
|
|
def_resp |
|
) |
| |
Value:
Initialize Write attribute command.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
direction | - direction of command (see zcl_frame_direction) |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_BY_TYPE
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_BY_TYPE |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
def_resp, |
|
|
|
write_attr_type |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_REQ_FRAME_CONTROL(cmd_ptr, def_resp); \
}
Initialize Write attribute command.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
def_resp | - enable/disable default response |
write_attr_type | - type of 'Write Attribute' command: default - |
- See also
- ZB_ZCL_CMD_WRITE_ATTRIB; no response -
-
ZB_ZCL_CMD_WRITE_ATTRIB_NO_RESP; undivided -
-
ZB_ZCL_CMD_WRITE_ATTRIB_UNDIV;
◆ ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_NO_RESP
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_NO_RESP |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
def_resp |
|
) |
| |
Value:
Initialize Write Attribute No Response command.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_UNDIV
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_UNDIV |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
def_resp |
|
) |
| |
Value:
Initialize Write Attribute Undivided command.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
def_resp | - enable/disable default response |
◆ ZB_ZCL_GENERAL_INIT_WRITE_ATTR_RESP
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_RESP |
( |
|
_buffer, |
|
|
|
_cmd_ptr, |
|
|
|
_seq |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_RESP_FRAME_CONTROL((_cmd_ptr)); \
}
Initialize Write attribute response command.
- Parameters
-
_buffer | - buffer to store command data |
_cmd_ptr | - pointer to a command data memory |
_seq | - command sequence |
◆ ZB_ZCL_GENERAL_INIT_WRITE_ATTR_RESP_EXT
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_RESP_EXT |
( |
|
_buffer, |
|
|
|
_cmd_ptr, |
|
|
|
_direction, |
|
|
|
_seq, |
|
|
|
_is_manuf, |
|
|
|
_manuf_id |
|
) |
| |
Value:{ \
ZB_ZCL_CONSTRUCT_GENERAL_COMMAND_RESP_FRAME_CONTROL_A((_cmd_ptr), (_direction), (_is_manuf)); \
}
Initialize Write attribute response command.
- Parameters
-
_buffer | - buffer to store command data |
_cmd_ptr | - pointer to a command data memory |
_direction | - direction of command (see zcl_frame_direction) |
_seq | - command sequence |
_is_manuf | - whether command is manufacturer specific |
_manuf_id | - manufacturer ID (needed if _is_manuf is set) |
◆ ZB_ZCL_GENERAL_SEND_WRITE_ATTR_REQ
#define ZB_ZCL_GENERAL_SEND_WRITE_ATTR_REQ |
( |
|
buffer, |
|
|
|
cmd_ptr, |
|
|
|
addr, |
|
|
|
dst_addr_mode, |
|
|
|
dst_ep, |
|
|
|
ep, |
|
|
|
profile_id, |
|
|
|
cluster_id, |
|
|
|
cb |
|
) |
| |
Value:{ \
ZB_ZCL_FINISH_PACKET(buffer, cmd_ptr) \
ZB_ZCL_SEND_COMMAND_SHORT(buffer, addr, dst_addr_mode, dst_ep, ep, profile_id, cluster_id, cb); \
}
Send Write attribute command.
- Parameters
-
buffer | - buffer to store command data |
cmd_ptr | - pointer to a command data memory |
addr | - address to send packet to |
dst_addr_mode | - addressing mode |
dst_ep | - destination endpoint |
ep | - sending endpoint |
profile_id | - profile identifier |
cluster_id | - cluster identifier |
cb | - callback for getting command send status |
◆ ZB_ZCL_GENERAL_SEND_WRITE_ATTR_RESP
#define ZB_ZCL_GENERAL_SEND_WRITE_ATTR_RESP |
( |
|
_buffer, |
|
|
|
_cmd_ptr, |
|
|
|
_addr, |
|
|
|
_dst_addr_mode, |
|
|
|
_dst_ep, |
|
|
|
_ep, |
|
|
|
_profile_id, |
|
|
|
_cluster_id, |
|
|
|
_cb |
|
) |
| |
Value:{ \
ZB_ZCL_FINISH_PACKET((_buffer), (_cmd_ptr)) \
ZB_ZCL_SEND_COMMAND_SHORT((_buffer), (_addr), (_dst_addr_mode), (_dst_ep), (_ep), \
(_profile_id), (_cluster_id), (_cb)); \
}
Send Write attribute response command.
- Parameters
-
_buffer | - buffer to store command data |
_cmd_ptr | - pointer to a command data memory |
_addr | - address to send packet to |
_dst_addr_mode | - addressing mode |
_dst_ep | - destination endpoint |
_ep | - sending endpoint |
_profile_id | - profile identifier |
_cluster_id | - cluster identifier |
_cb | - callback for getting command send status |
◆ ZB_ZCL_GENERAL_SEND_WRITE_ATTRS_REQ
#define ZB_ZCL_GENERAL_SEND_WRITE_ATTRS_REQ |
( |
|
buffer, |
|
|
|
addr, |
|
|
|
addr_mode, |
|
|
|
dst_ep, |
|
|
|
ep, |
|
|
|
prof_id, |
|
|
|
cluster_id |
|
) |
| |
Value: ZB_ZCL_SEND_GENERAL_COMMAND_REQ_SHORT( \
Send "write attributes" request. deprecate.
◆ ZB_ZCL_GENERAL_SUCCESS_WRITE_ATTR_RESP
#define ZB_ZCL_GENERAL_SUCCESS_WRITE_ATTR_RESP |
( |
|
_cmd_ptr | ) |
|
Value:
Add Success status value to Write attribute response command payload.
- Parameters
-
_cmd_ptr | - pointer to a command data memory |
◆ ZB_ZCL_GET_NEXT_WRITE_ATTR_RES
#define ZB_ZCL_GET_NEXT_WRITE_ATTR_RES |
( |
|
data_buf, |
|
|
|
write_attr_res |
|
) |
| |
Parses Write attribute response and returns next Write attribute status or NULL if there is no more data.
If response contains invalid data, NULL is returned.
- Parameters
-
data_buf | - ID zb_bufid_t of a buffer containing write attribute response data |
write_attr_res | - out pointer to zb_zcl_write_attr_res_t, containing Write attribute status |
- Note
- data_buf buffer should contain Write attribute response payload, without ZCL header. Each parsed Write attribute response is extracted from initial data_buf buffer
◆ zb_zcl_write_attr_req_t
ZCL Write Attribute Command frame.
- See also
- ZCL spec, 2.4.3 Write Attributes Command
◆ zb_zcl_write_attr_res_t
ZCL Write Attribute Command frame.
- See also
- ZCL spec, 2.4.3 Write Attributes Command
#define ZB_AF_HA_PROFILE_ID
Definition: zboss_api_af.h:287
#define ZB_ZCL_START_PACKET(zbbuf)
Initializes zb_buf_t buffer and returns pointer to the beginning of array.
Definition: zb_zcl_common.h:1428
#define ZB_ZCL_CLUSTER_ID_ON_OFF
Definition: zb_zcl_common.h:211
#define ZB_ZCL_ENABLE_DEFAULT_RESPONSE
Definition: zb_zcl_common.h:935
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ_BY_TYPE(buffer, cmd_ptr, def_resp, write_attr_type)
Initialize Write attribute command.
Definition: zb_zcl_commands.h:958
#define ZB_ZCL_CMD_WRITE_ATTRIB_RESP
Definition: zb_zcl_commands.h:70
#define ZB_ZCL_GET_SEQ_NUM()
Return next sequence number for ZCL frame.
Definition: zb_zcl_common.h:1348
#define ZB_ZCL_NOT_MANUFACTURER_SPECIFIC
Definition: zb_zcl_common.h:911
#define ZB_ZCL_CMD_WRITE_ATTRIB_UNDIV
Definition: zb_zcl_commands.h:69
#define ZB_ZCL_CMD_WRITE_ATTRIB
Definition: zb_zcl_commands.h:68
#define ZB_ZCL_CMD_WRITE_ATTRIB_NO_RESP
Definition: zb_zcl_commands.h:71
#define ZB_ZCL_GENERAL_SEND_WRITE_ATTR_REQ( buffer, cmd_ptr, addr, dst_addr_mode, dst_ep, ep, profile_id, cluster_id, cb)
Send Write attribute command.
Definition: zb_zcl_commands.h:1031
#define ZB_ZCL_GENERAL_INIT_WRITE_ATTR_REQ(buffer, cmd_ptr, def_resp)
Initialize Write attribute command.
Definition: zb_zcl_commands.h:970
ZCL Write Attribute Command frame.
Definition: zb_zcl_commands.h:830
#define ZB_APS_ADDR_MODE_16_ENDP_PRESENT
Definition: zboss_api_aps.h:107
#define ZB_ZCL_GENERAL_ADD_VALUE_WRITE_ATTR_REQ(cmd_ptr, attr_id, attr_type, attr_val)
Add attribute value to command payload.
Definition: zb_zcl_commands.h:1013
zb_uint8_t * zb_zcl_put_value_to_packet(zb_uint8_t *cmd_ptr, zb_uint8_t attr_type, zb_uint8_t *attr_value)
#define ZB_ZCL_GENERAL_GET_NEXT_WRITE_ATTR_REQ(data_ptr, data_len, write_attr_req)
Parses Write attribute request and returns next Write attribute record or NULL if there is no more da...
Definition: zb_zcl_commands.h:856
#define ZB_ZCL_STATUS_SUCCESS
Definition: zb_zcl_common.h:346