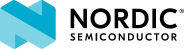 |
nrfxlib API 2.8.99
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
40#ifndef NRF_802154_PERIPHERALS_NRF53_H__
41#define NRF_802154_PERIPHERALS_NRF53_H__
46#include "nrf_802154_sl_periphs.h"
59#ifndef NRF_802154_EGU_INSTANCE_NO
60#define NRF_802154_EGU_INSTANCE_NO 0
72#define NRF_802154_EGU_INSTANCE NRFX_CONCAT_2(NRF_EGU, NRF_802154_EGU_INSTANCE_NO)
82#define NRF_802154_EGU_IRQ_HANDLER \
83 NRFX_CONCAT_3(EGU, NRF_802154_EGU_INSTANCE_NO, _IRQHandler)
90#ifndef NRF_802154_EGU_USED_MASK
91#define NRF_802154_EGU_USED_MASK (1 << NRF_802154_EGU_INSTANCE_NO)
100#ifndef NRF_802154_RTC_INSTANCE_NO
101#define NRF_802154_RTC_INSTANCE_NO 2
110#define NRF_802154_DPPIC_INSTANCE NRF_DPPIC
120#ifndef NRF_802154_DPPI_RADIO_DISABLED
121#define NRF_802154_DPPI_RADIO_DISABLED 7U
132#ifndef NRF_802154_DPPI_RADIO_READY
133#define NRF_802154_DPPI_RADIO_READY 4U
144#ifndef NRF_802154_DPPI_RADIO_ADDRESS
145#define NRF_802154_DPPI_RADIO_ADDRESS 5U
156#ifndef NRF_802154_DPPI_RADIO_END
157#define NRF_802154_DPPI_RADIO_END 6U
168#ifndef NRF_802154_DPPI_RADIO_PHYEND
169#define NRF_802154_DPPI_RADIO_PHYEND 8U
181#ifndef NRF_802154_DPPI_EGU_TO_RADIO_RAMP_UP
182#define NRF_802154_DPPI_EGU_TO_RADIO_RAMP_UP 10U
194#ifndef NRF_802154_DPPI_TIMER_COMPARE_TO_RADIO_TXEN
195#define NRF_802154_DPPI_TIMER_COMPARE_TO_RADIO_TXEN 10U
205#ifndef NRF_802154_DPPI_RADIO_SYNC_TO_EGU_SYNC
206#define NRF_802154_DPPI_RADIO_SYNC_TO_EGU_SYNC 12U
214#ifndef NRF_802154_DPPI_RADIO_CCAIDLE
215#define NRF_802154_DPPI_RADIO_CCAIDLE 9U
223#ifndef NRF_802154_DPPI_RADIO_CCABUSY
224#define NRF_802154_DPPI_RADIO_CCABUSY 3U
232#define NRF_802154_DPPI_RADIO_TEST_MODE_USED_MASK 0U
239#ifndef NRF_802154_DPPI_RADIO_HW_TRIGGER
240#define NRF_802154_DPPI_RADIO_HW_TRIGGER 15U
248#ifndef NRF_802154_DPPI_CHANNELS_USED_MASK
249#define NRF_802154_DPPI_CHANNELS_USED_MASK ( \
250 (1UL << NRF_802154_DPPI_RADIO_DISABLED) | \
251 (1UL << NRF_802154_DPPI_RADIO_READY) | \
252 (1UL << NRF_802154_DPPI_RADIO_ADDRESS) | \
253 (1UL << NRF_802154_DPPI_RADIO_END) | \
254 (1UL << NRF_802154_DPPI_RADIO_PHYEND) | \
255 (1UL << NRF_802154_DPPI_EGU_TO_RADIO_RAMP_UP) | \
256 (1UL << NRF_802154_DPPI_TIMER_COMPARE_TO_RADIO_TXEN) | \
257 (1UL << NRF_802154_DPPI_RADIO_SYNC_TO_EGU_SYNC) | \
258 (1UL << NRF_802154_DPPI_RADIO_CCAIDLE) | \
259 (1UL << NRF_802154_DPPI_RADIO_CCABUSY) | \
260 (1UL << NRF_802154_DPPI_RADIO_HW_TRIGGER) | \
261 NRF_802154_DPPI_RADIO_TEST_MODE_USED_MASK | \
262 NRF_802154_SL_PPI_CHANNELS_USED_MASK)
270#ifndef NRF_802154_DPPI_GROUPS_USED_MASK
271#define NRF_802154_DPPI_GROUPS_USED_MASK 0UL