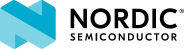 |
nrfxlib API 2.8.99
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
40#ifndef NRF_802154_PERIPHERALS_NRF52_H__
41#define NRF_802154_PERIPHERALS_NRF52_H__
46#include "hal/nrf_ppi.h"
47#include "nrf_802154_sl_periphs.h"
60#ifndef NRF_802154_EGU_INSTANCE_NO
62#define NRF_802154_EGU_INSTANCE_NO 0
75#define NRF_802154_EGU_INSTANCE NRFX_CONCAT_2(NRF_EGU, NRF_802154_EGU_INSTANCE_NO)
85#define NRF_802154_EGU_IRQ_HANDLER \
86 NRFX_CONCAT_3(NRFX_CONCAT_3(SWI, NRF_802154_EGU_INSTANCE_NO, _EGU), \
87 NRF_802154_EGU_INSTANCE_NO, \
96#ifndef NRF_802154_RTC_INSTANCE_NO
98#define NRF_802154_RTC_INSTANCE_NO 2
115#ifndef NRF_802154_PPI_RADIO_RAMP_UP_TRIGG
116#define NRF_802154_PPI_RADIO_RAMP_UP_TRIGG NRF_PPI_CHANNEL6
128#ifndef NRF_802154_PPI_RADIO_DISABLED_TO_EGU
129#define NRF_802154_PPI_RADIO_DISABLED_TO_EGU NRF_PPI_CHANNEL6
140#ifndef NRF_802154_PPI_EGU_TO_RADIO_RAMP_UP
141#define NRF_802154_PPI_EGU_TO_RADIO_RAMP_UP NRF_PPI_CHANNEL7
152#ifndef NRF_802154_PPI_EGU_TO_TIMER_START
153#define NRF_802154_PPI_EGU_TO_TIMER_START NRF_PPI_CHANNEL8
166#ifndef NRF_802154_PPI_RADIO_CRCERROR_TO_TIMER_CLEAR
167#define NRF_802154_PPI_RADIO_CRCERROR_TO_TIMER_CLEAR NRF_PPI_CHANNEL9
180#ifndef NRF_802154_PPI_RADIO_CCAIDLE_TO_FEM_GPIOTE
181#define NRF_802154_PPI_RADIO_CCAIDLE_TO_FEM_GPIOTE NRF_PPI_CHANNEL9
194#ifndef NRF_802154_PPI_TIMER_COMPARE_TO_RADIO_TXEN
195#define NRF_802154_PPI_TIMER_COMPARE_TO_RADIO_TXEN NRF_PPI_CHANNEL9
207#ifndef NRF_802154_PPI_RADIO_CCABUSY_TO_RADIO_CCASTART
208#define NRF_802154_PPI_RADIO_CCABUSY_TO_RADIO_CCASTART NRF_PPI_CHANNEL10
218#ifndef NRF_802154_PPI_RADIO_SYNC_TO_EGU_SYNC
219#define NRF_802154_PPI_RADIO_SYNC_TO_EGU_SYNC NRF_PPI_CHANNEL11
222#define NRF_802154_DISABLE_BCC_MATCHING_PPI_CHANNELS_USED_MASK \
223 (1 << NRF_802154_PPI_RADIO_SYNC_TO_EGU_SYNC)
233#ifndef NRF_802154_PPI_CORE_GROUP
234#define NRF_802154_PPI_CORE_GROUP NRF_PPI_CHANNEL_GROUP0
243#ifndef NRF_802154_PPI_ABORT_GROUP
244#define NRF_802154_PPI_ABORT_GROUP NRF_PPI_CHANNEL_GROUP1
252#ifndef NRF_802154_EGU_USED_MASK
253#define NRF_802154_EGU_USED_MASK (1 << NRF_802154_EGU_INSTANCE_NO)
261#ifndef NRF_802154_PPI_CHANNELS_USED_MASK
262#define NRF_802154_PPI_CHANNELS_USED_MASK ((1 << NRF_802154_PPI_RADIO_DISABLED_TO_EGU) | \
263 (1 << NRF_802154_PPI_RADIO_RAMP_UP_TRIGG) | \
264 (1 << NRF_802154_PPI_EGU_TO_RADIO_RAMP_UP) | \
265 (1 << NRF_802154_PPI_EGU_TO_TIMER_START) | \
266 (1 << NRF_802154_PPI_RADIO_CRCERROR_TO_TIMER_CLEAR) | \
267 (1 << NRF_802154_PPI_RADIO_CCAIDLE_TO_FEM_GPIOTE) | \
268 (1 << NRF_802154_PPI_TIMER_COMPARE_TO_RADIO_TXEN) | \
269 (1 << NRF_802154_PPI_RADIO_CCABUSY_TO_RADIO_CCASTART) | \
270 NRF_802154_DISABLE_BCC_MATCHING_PPI_CHANNELS_USED_MASK | \
271 NRF_802154_SL_PPI_CHANNELS_USED_MASK | \
272 NRF_802154_DEBUG_PPI_CHANNELS_USED_MASK)
280#ifndef NRF_802154_PPI_GROUPS_USED_MASK
281#define NRF_802154_PPI_GROUPS_USED_MASK ((1 << NRF_802154_PPI_CORE_GROUP) | \
282 (1 << NRF_802154_PPI_ABORT_GROUP) )